The WordPress Playground and the plugin preview functionality are great features that enhance the WordPress experience for plugin users.
With the upcoming WordPress 6.5 release, I took the opportunity to update my plugins and add a demo/preview functionality using a new feature in the WordPress plugin directory – the WordPress Playground.
The plugins I updated are “Good-Slider” and “Shareable Password Protected Posts“. Both are small plugins, making the demo setup quick and straightforward. And I think the preview is a neat feature, because the plugins’ functionality can be easily demonstrated and explained.
What’s “WordPress Playground”?
The WordPress Playground is a project that allows you to run WordPress directly in your browser by using PHP-WASM. It eliminates the need for a database or a PHP server, making WordPress accessible from any device without login.
In the last few months I found myself using it to test Gutenberg PRs, quick testing of plugins or testing some WordPress core PRs on different WordPress/PHP versions.
It’s amazing that the whole application can run in your browser, and the technology behind it can even power mobile apps and interactive code blocks.
In encourage you to try it out and play with it: playground.wordpress.net
Documentation: https://wordpress.github.io/wordpress-playground
WordPress Plugin Directory Preview Function
With WordPress Playground plugin developers can provide simple demos and previews of their plugins for potential users, without the user having to install and set up the plugin.
After some pushback, the feature was implemented as opt-in. And I think that’s good, because it doesn’t work for all type of plugins (e.g. plugins depending on WooCommerce, a real database, …).
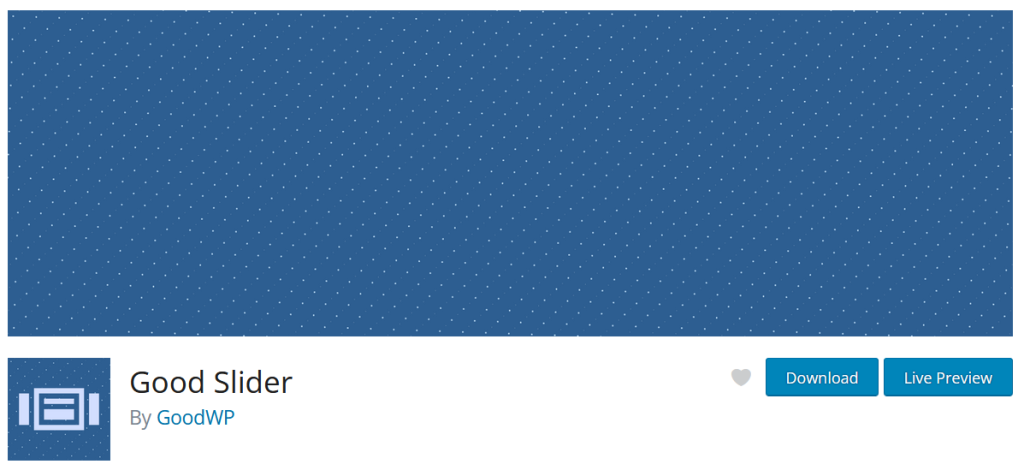
When a user wants to test the plugin and make sure it meets their expectations, they can just click “Live Preview” and a new WordPress Playground instance opens. The instance has pre-defined plugins, settings and contents based on the blueprint.
Implementing it in your plugin
Adding the preview to your plugin is actually pretty straight-forward:
- Add a
blueprints/blueprint.json
to yourassets
directory - Test the preview on your plugins page in the repository (only you can see it)
- Enable the preview functionality for all users (In the advanced view of your plugin)
The hard part is actually building the blueprint to provide users a demo environment which shows your plugins functionality well.
Blueprint.json Format for WordPress Playground
The blueprint.json
file is used to set up a WordPress Playground instance. It can specify things like PHP and WP versions, the landing page, and a series of automated steps such as logging in, and installing and activating plugins and themes, importing content and setting options.
{
"$schema": "https://playground.wordpress.net/blueprint-schema.json",
"landingPage": "/wp-admin/",
"preferredVersions": {
"php": "8.0",
"wp": "latest"
},
"phpExtensionBundles": ["kitchen-sink"],
"steps": [
{
"step": "login",
"username": "admin",
"password": "password"
}
]
}
This example blueprint will create a WordPress instance with PHP 8.0, the latest core version and log you into wp-admin.
You can find the full schema and options about the blueprint format in the WordPress Playground documentation.
Creating and testing your blueprint
Developing and testing the blueprint can be a bit cumbersome. There are a few methods which I will describe below. Unfortunately, I had to use a combination of them and also push a first draft to GitHub and then test and refine it from there.
Using an iframe + JavaScript API
You can create a Playground instance via iframe and use its JavaScript API. I created a test.html
with the iframe and “developed” the blueprint inside of it:
<iframe id="wp-playground" style="width: 1200px; height: 800px"></iframe>
<script type="module">
import { startPlaygroundWeb } from 'https://playground.wordpress.net/client/index.js';
const client = await startPlaygroundWeb({
iframe: document.getElementById('wp-playground'),
remoteUrl: `https://playground.wordpress.net/remote.html`,
blueprint: {
landingPage: '/wp-admin/',
preferredVersions: {
php: '8.0',
wp: 'latest',
},
steps: [
{
step: 'login',
username: 'admin',
password: 'password',
},
{
step: 'installPlugin',
pluginZipFile: {
resource: 'wordpress.org/plugins',
slug: 'good-slider',
},
},
],
},
});
</script>
Using wp-now
wp-now is another tool to run WordPress Playground via npm locally. It supports passing a blueprint to initialize. Which would be perfect for testing, but unfortunately I kept getting errors (include(wordpress/wp-load.php): Failed to open stream: No such file or directory
on the setSiteOptions
step and DOMParser is not defined
on the installPlugin
step). So that didn’t work. But I will try this method again in the future. It should be pretty useful.1
Using the hosted Playground with a blueprint
The hosted instance of WordPress playground at playground.wordpress.net allows passing a blueprint-url
parameter. So if you already have your blueprint hosted somewhere, you can use this method. For example: https://playground.wordpress.net/?blueprint-url=https://raw.githubusercontent.com/goodwp/good-slider/main/.wordpress-org/blueprints/blueprint.json
A problem I ran into a couple of times was CORS. I wanted to load a blueprint from somewhere (in this case just my web server) or load the demo content (see below), but since the resources are fetched client-side via fetch
, you have to set the correct CORS headers when serving the file. Uploading the file to GitHub and accessing it via its raw.githubusercontent.com
URL is actually the easiest way 😅.
Adding Steps
In my preview, I just installed my plugin, set some common site options (a name, the default theme) and then loaded some demo contents.
The landingPage
property is the URL the user will get to after all steps are done. You could use a settings page, the edit-screen of a post or the frontend of the site.
Adding demo content
I wanted to show my plugin in action by providing some demo content. Therefore, I built the demo content in my development environment and exported it via the WordPress export tool. One step in the blueprint then is to import this XML.
I uploaded the example data to GitHub and imported it from there. If you’re developing the blueprint.json
and have not uploaded a demo content, you have to guess the URL, but it’s pretty simple based on where you upload it. In my case, it is https://raw.githubusercontent.com/goodwp/good-slider/main/.wordpress-org/blueprints/demo-content.xml
.
The post ID from the import is important because, as a landing page of the blueprint, I defined the edit screen of this post.
As far as I can see, many other plugins are doing it like this way. So I guess it’s the way to go. The blueprint documentation shows examples of running a wp_insert_post
PHP code, but you would have to write that code yourself. I wanted to create real examples of my block in the editor and then export them – and for block plugins I think that’s the best way.
The final blueprint
{
"$schema": "https://playground.wordpress.net/blueprint-schema.json",
"preferredVersions": {
"php": "8.1",
"wp": "6.4"
},
"features": {
"networking": true
},
"phpExtensionBundles": [ "kitchen-sink" ],
"landingPage": "/wp-admin/post.php?post=8&action=edit",
"steps": [
{
"step": "login",
"username": "admin",
"password": "password"
},
{
"step": "setSiteOptions",
"options": {
"blogname": "Good Slider Demo",
"blog_charset": "UTF-8",
"template": "twentytwentyfour",
"stylesheet": "twentytwentyfour"
}
},
{
"step": "installPlugin",
"pluginZipFile": {
"resource": "wordpress.org/plugins",
"slug": "good-slider"
},
"options": {
"activate": true
}
},
{
"step": "importFile",
"file": {
"resource": "url",
"url": "https://raw.githubusercontent.com/goodwp/good-slider/main/.wordpress-org/blueprints/demo-content.xml"
}
}
]
}
That’s the final and full version of my blueprint for the Good Slider plugin. You can see the source code on GitHub. And you can try out the preview from the plugin repository or with this link: https://wordpress.org/plugins/good-slider/?preview=1
Conclusion
The WordPress Playground and the Preview functionality in the WordPress Plugin Repository are game-changing features that enhance the WordPress experience. They offer a seamless, user-friendly platform for experimenting, learning, and showcasing WordPress capabilities. Whether you’re a beginner exploring WordPress or a developer testing a new plugin, these features offer a convenient and efficient way to work with WordPress.
Links:
- Feature announcement: https://make.wordpress.org/meta/2023/11/22/plugin-directory-preview-button-revisited
- Preview documentation: https://developer.wordpress.org/plugins/wordpress-org/previews-and-blueprints/
- Playground documentation: https://wordpress.github.io/wordpress-playground/
- I’ve created an issue report on GitHub. ↩︎